The focus we are using in this series of tutorial is from Tkinter, or known as TK.
from Tkinter import * root = Tk() # Change the background color to light green root['bg'] = 'light green' # Create a title widget in the frame of root simpleTitle = Label(root) simpleTitle['text'] = 'Hello Tkinter!' simpleTitle.pack() mainloop()The output of the program above is like this, it is a simple window with the string, 'Hello Tkinter!', but try to make the window size better!
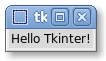
Do you see the green color? That's the light green background color we set. It is not shown when the GUI launched, you can resize the window, by moving your mouse pointer to the border of the window, and then just drag it larger. So there you have it you see the background color. You can also change the color of the text label if you want it too.
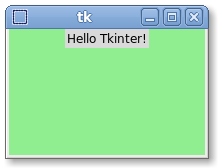
Look at line 1:
from Tkinter import *We have to import the module of Tkinter, because although python does ship with this, you have to let python know you want to use this.
We create a Tk() object named root, this is like a base frame. And then we can change the background color, by accessing its ['bg'] indexed variable, and assign it to 'light green'. If you want, you can change it to 'light pink' as well.
Look at line 8:
simpleTitle = Label(root) simpleTitle['text'] = 'Hello Tkinter!' simpleTitle.pack()We here create a Label named simpleLabel, it is based on the root's frame, that's the reason. If there is another frame, we would replace root with the name of the frame. For example: myLabel = Label(anotherFrame). Just like ['bg'] field, there is a ['text'] field associate with it too, we access it the same way we access the background color, we can assign a string to it. And at last, we have to call .pack(), to ask Tk to draw it onto the screen. When we make changes, we have to always do .pack(). Notice pack() is a function, it actually takes parameters!
Reference: http://docs.python.org/library/tkinter.html#packer-options
Here is the list of possible options:
- expand: if it is set to true, it will expand as the window's size get bigger. Ex: foo.pack(expand=YES)
- fill: it is to screen, there are 3 options to it, either X, Y, BOTH
Ex: foo.pack(fill=BOTH)- side: it get where your widget want to be positioned. TOP (default), BOTTOM, LEFT, RIGHT
Ex: foo.pack(side=LEFT)- anchor: which way it snaps on to, it is think as a compass, has 8 directions. "n", "ne", "e", "se", "s", "sw", "w", "nw", "center"
Ex: foo.pack(anchor=w)
Example of such chained options:
foo.pack(expand=YES, side=LEFT, fill=X)
i happen need to take a crash course in python. so i am test driving your tutorials. i noticed a lot of grammatical awkwardness in the text. what is the best method you would like me to propose edits?
ReplyDelete- Alice
How about email me your gmail account? Either here or on facebook. I'll add you as author as well.
ReplyDelete- George