// A game that plays rock paper scissor with user import javax.swing.JOptionPane; public class GameRockPaperScissor { public static void main(String[] args) { // Prompt the user to input the option they choose String humanInput = JOptionPane.showInputDialog( "Enter rock, paper, or scissor:"); // Convert to lower case humanInput = humanInput.toLowerCase(); // Generate a random number // Scissor (0), rock (1), paper (2) int random = (int)(Math.random() * 3); String computerInput; // Computer's choice if (random == 0) computerInput = "rock"; else if (random == 1) computerInput = "paper"; else computerInput = "scissor"; String message = "The computer is " + computerInput + ". You are " + humanInput; // Determine who win boolean isTie = computerInput.equals(humanInput); boolean isWin = ((computerInput.equals("rock") && humanInput.equals("scissor")) || (computerInput.equals("paper") && humanInput.equals("rock")) || (computerInput.equals("scissor") && humanInput.equals("paper"))); // Prepare the message if (isWin) message += ". Computer Won."; else if (isTie) message += " too. It is Tie"; // There are 3 options, computer win, tie, or you win // So we don't have to test here, if the code reach here, human win. else message += ". You Won"; // Display the result on the screen JOptionPane.showMessageDialog(null, message); } }
George Chan's Blog
My notes on Python and C++, import this.
Sunday, June 30, 2013
Java: Rock, Paper, Scissor Game Source Code
This is the source code for how to implement a rock, paper, scissor game in JAVA. It has a swing GUI interface (using JOptionPane) that prompt the user for input.
Labels:
game,
GUI,
java,
JOptionPane,
paper,
program,
project,
rock,
scissor,
source,
Source Code,
swing,
ygchan89
Thursday, May 31, 2012
Blog Index
I have been writing tutorials and posting different projects for a while now, I decided to write an index for easy look up for the different post. They are divided to a few section as the follow. I hope you can find what you are looking for, if not, try to use the search feature located in the lower right side. If you have an question, please feel free to email me or leave me a message in the comment.
Python Tutorial
C++ Tutorial
Python Tutorial
- Python Reference
A list of useful website and textbook review - csci133c1.py Basic Hello World, declare variables, simple for loop control statement
- csci133c2.py Nested for loops, list, counting, if statement
- csci133c3.py Introduction to function, string's class function, useful tip for handling string
- csci133c4.py Opening a file, and using everything we know together. Additional to a brief review practice problem, introduce dictionary
- csci133c5.py
Talks about function, what are they, how to define them - csci133c6.py
A taste of python's GUI, Tkinter. Introducing the title, frame, button, input widget, and what they can do with each other - csci133buildin.py
Discuss what are the build-in data type (Object) in Python - csci133number.py
Document Integer, Float, Long, and Complex number - csci133class.py
Some notes I have on python's class, tkinter's other module - csci133ifelif
Demonstration of the uses of if and elif (else-if) keyword - csci133c7.py
Classic how to clean up a string and storing the string's corresponding line numbers, it discuss the use all the things we learned so far - csci133c8.py
Some notes on the python's class, and subclass too - csci133allCombination.py
Example of why nest while loop is bad, and how to use one if you really must have it. Plus counter solutions. - csci133rep1.py
An example of how to validate input of telephone number in a specific format, demonstrating simple use of regular expression in python. - csci133averageGPA.py
Simple exercise program to calculate the grade point average - csci133rockPaperScissors.py
A game of rock, paper, scissor implemented in python - csci133clock.py
An example of how to make a clock with tkinter in Python - csci133timer.py
A tutorial on how to build a timer with tkinter in Python
Stackoverflow Goodies
- Python: Get the most frequent elements from list when there is more than one
Given a list that is unsorted, how do you get the most frequent appeared element? - Python: Regular Expression 101 Example Code
A very simple demonstration of Python's Regular Expression, in particular, how to validate if a telephone number is in the format we want xxx-xxx-xxxx - Python: How to print the ValueError Error Message
It is actually better to expect error than to test them out - Python: [] vs. {} vs. ()
A flash card for: list vs. dictionary / set vs. tuple - Python Tkinter: How to change window size without using canvas
Use the .pack_propagate() method to change the flag - Python: When NOT To use Global Variable, Use Return!
Why you might not want to use global variable all the time, and what do you do with returned values. - Python: How to remove, or pop an element randomly from a list
Use randrange() instead of random.choice(range(myList)) - Python: How to search a list of class element by their data
Put the _classVariable into a dictinoary {} into your class - Python: How to find the integer and float in a string
If you given a string in the form of an equation, how to extract the integer and float from the string. Beginner style! No regular expression used. - Python: How to insert characters to the string at the end
Example of 3 different way you can insert some characters into the string - Python: How to load image to tkinter window example (GIF only)
Example to how to load an image and display on the tkinter
C++ Tutorial
- C++ Resource Discussion about what are the good reference for the class
- csci135c1.cpp Hello world, talks about cout and endl
- csci135c2.cpp More cout, and for loop
- csci135c3.cpp while loops and do while loop tutorial in great details
Tuesday, May 22, 2012
Python: How to make a stop watch timer using tkinter
First thing we have to understand is how the time actually work. If you do, you can skip ahead to the next section. I was not completely sure how the time work. I confirmed from the search on google and here is what it said.
We use the similar code as the Clock, there is a Lable (a textbox) called timeText, and we will update it every 1 centisecond, and increment our time sturcture by 1 as well. Take a look at the way it is designed.
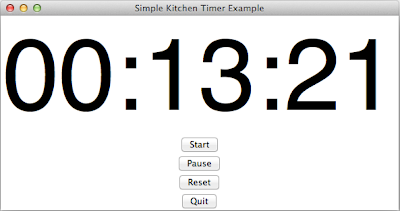
The following program contains 4 buttons (start, pause, reset, quit). Which they do what they are named after respectly. The reset function work both running and paused clock. Maybe it is a good idea to add a time lap feature?
1 min = 60 seconds 1 seconds = 100 centiseconds
We use the similar code as the Clock, there is a Lable (a textbox) called timeText, and we will update it every 1 centisecond, and increment our time sturcture by 1 as well. Take a look at the way it is designed.
# timer is a list of integer, in the following order timer = [minutes, seconds, centiseconds]Notice in the actual code it is initialized to 0,0,0. But it then created a problem of not being show as 00:00:00 as a normal stop watch would. One way to get around this is to use the string's format.() function.
pattern = '{0:02d}:{1:02d}:{2:02d}' timeString = pattern.format(timer[0], timer[1], timer[2]){0:02d}, the first 0 means which parameter in the format() function call, 02d means, it is expecting an integer of length 2, the 02 means it is padding with leading zeros infront of it. Take note of this feature, as it is really powerful, you can mix and match different parameters, instead of {0} {1} {2}, you can do creative things like {2} {1} {1} {2} {0}, (such as different order, multiple use of the same varaible, etc).
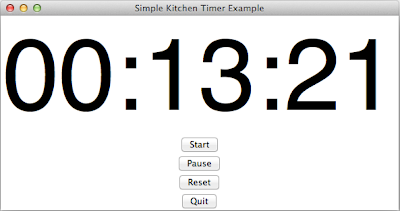
The following program contains 4 buttons (start, pause, reset, quit). Which they do what they are named after respectly. The reset function work both running and paused clock. Maybe it is a good idea to add a time lap feature?
import Tkinter as tk # Note: Python 2.6 or higher is required for .format() to work def update_timeText(): if (state): global timer # Every time this function is called, # we will increment 1 centisecond (1/100 of a second) timer[2] += 1 # Every 100 centisecond is equal to 1 second if (timer[2] >= 100): timer[2] = 0 timer[1] += 1 # Every 60 seconds is equal to 1 min if (timer[1] >= 60): timer[0] += 1 timer[1] = 0 # We create our time string here timeString = pattern.format(timer[0], timer[1], timer[2]) # Update the timeText Label box with the current time timeText.configure(text=timeString) # Call the update_timeText() function after 1 centisecond root.after(10, update_timeText) # To start the kitchen timer def start(): global state state = True # To pause the kitchen timer def pause(): global state state = False # To reset the timer to 00:00:00 def reset(): global timer timer = [0, 0, 0] timeText.configure(text='00:00:00') # To exist our program def exist(): root.destroy() # Simple status flag # False mean the timer is not running # True means the timer is running (counting) state = False root = tk.Tk() root.wm_title('Simple Kitchen Timer Example') # Our time structure [min, sec, centsec] timer = [0, 0, 0] # The format is padding all the pattern = '{0:02d}:{1:02d}:{2:02d}' # Create a timeText Label (a text box) timeText = tk.Label(root, text="00:00:00", font=("Helvetica", 150)) timeText.pack() startButton = tk.Button(root, text='Start', command=start) startButton.pack() pauseButton = tk.Button(root, text='Pause', command=pause) pauseButton.pack() resetButton = tk.Button(root, text='Reset', command=reset) resetButton.pack() quitButton = tk.Button(root, text='Quit', command=exist) quitButton.pack() update_timeText() root.mainloop()
Python: How to make a clock in tkinter using time.strftime
This is a tutorial on how to create a clock / Timer using tkinter in Python, note you might have to change Tkinter to tkinter depending on your version of the Python you have. I am using Python 3.2 at the moment. The code itself is quite simple, the only part you need to know is how to get the current time, using time.strftime. You can also get the day of the week, the current month, etc, by changing the parameter you are supplying the function.
(See the link for the full table of possible options). But yes, almost anything you can think of any use of, it is there already. :) So this can actual be developed to a calendar like GUI.
Related Tutorial with source code
Stop watch GUI (for counting time): http://ygchan.blogspot.com/2012/05/python-stop-watch-timer-source-code.html
Reference: http://docs.python.org/library/string.html#formatstrings
Related Tutorial with source code
Stop watch GUI (for counting time): http://ygchan.blogspot.com/2012/05/python-stop-watch-timer-source-code.html
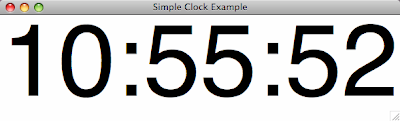
import Tkinter as tk import time def update_timeText(): # Get the current time, note you can change the format as you wish current = time.strftime("%H:%M:%S") # Update the timeText Label box with the current time timeText.configure(text=current) # Call the update_timeText() function after 1 second root.after(1000, update_timeText) root = tk.Tk() root.wm_title("Simple Clock Example") # Create a timeText Label (a text box) timeText = tk.Label(root, text="", font=("Helvetica", 150)) timeText.pack() update_timeText() root.mainloop()Reference: http://docs.python.org/library/time.html#time.strftime
Reference: http://docs.python.org/library/string.html#formatstrings
Sunday, May 20, 2012
Python: How to load and display an image (GIF) in tkinter
Reference: http://stackoverflow.com/q/10675551/1276534
Credits: mg_, Carlos, George, Bryan Oakley
This is a simple code that get the input from the
inputBox
to myText,
and also display a picture (gif only) to the tkinter window. Depending on what
else you need to check or do, you can add more functions to it. Notice
you might have to play around with the order of the line image = tk.PhotoImage(data=b64_data)
. Because if you put it right after b64_data = ...
.
It will gives you error. See reference at the
bottom if you want to learn more.import tkinter as tk import urllib.request import base64 # Download the image using urllib URL = "http://www.contentmanagement365.com/Content/Exhibition6/Files/369a0147-0853-4bb0-85ff-c1beda37c3db/apple_logo_50x50.gif" u = urllib.request.urlopen(URL) raw_data = u.read() u.close() b64_data = base64.encodestring(raw_data) # The string you want to returned is somewhere outside myText = 'empty' def getText(): global myText # You can perform check on some condition if you want to # If it is okay, then store the value, and exist myText = inputBox.get() print('User Entered:', myText) root.destroy() root = tk.Tk() # Just a simple title simpleTitle = tk.Label(root) simpleTitle['text'] = 'Please enter your input here' simpleTitle.pack() # The image (but in the label widget) image = tk.PhotoImage(data=b64_data) imageLabel = tk.Label(image=image) imageLabel.pack() # The entry box widget inputBox = tk.Entry(root) inputBox.pack() # The button widget button = tk.Button(root, text='Submit', command=getText) button.pack() tk.mainloop()
Here is the reference if you want to know more about the Tkinter Entry Widget: http://effbot.org/tkinterbook/entry.htm
Reference on how to get the image: Stackoverflow Question
Friday, May 11, 2012
Python: Rock, Paper, Scissor Game Source Code
I implemented a simple game of rock, paper, scissors game in python as a after school project. The game itself is really simple, not that I need to. But I pay a quick visit to wiki's page, and read through it. The original program has a long list of if statement, but as I was thinking through it, it comes to me quickly, that you actually does not need to have that many control statement, just three is enough. Since there is only three possible outcome.
The logic goes like this:
The logic goes like this:
- If you and the computer's outcome (choice) is the same, then you are tie. Regardless of what kind of choice you or your computer made. A tie is a tie.
- Then there is 3 possible ways you can win.
- You are Rock, computer is Scissors
- You are Paper, computer is Rock
- You are Scissors, computer is Paper
- If you didn't tie, and you didn't win, your only option is to lose the game.
# Hunter College CSCI133 Python Project # Rock Paper Scissors Game Source code in Python # Implemented by George Chan, 5/2/2012 # Email: ygchan89@gmail.com # A simple game to play rock, paper, scissors with the computer import random # Create the list of of choices, which stored at string gameChoices = ['rock', 'paper', 'scissors'] # Our default ready flag is false ready = False print('rock, paper, scissors Game!') while (not ready): myResponse = raw_input('Please enter which option you are going with: ') myResponse = myResponse.lower() # We will change our flag to true and break out of the loop # if and only if the input is equiv to one of our choices if (myResponse in gameChoices): ready = True # Randomly pick a choice from the list gameChoices computerResponse = random.choice(gameChoices) print('Computer picked:', computerResponse) # There are three possible condition of the game, either you tie, # with the computer or you win, else you lose # Tie condition is when you and computer choice is the same if (myResponse == computerResponse): print('Tie Game') # Win condition is the below three possible one elif (myResponse == 'rock' and computerResponse == 'scissors' or myResponse == 'paper' and computerResponse == 'rock' or myResponse == 'scissors' and computerResponse == 'paper'): print('You win') # If you didn't tie, didn't win, then you must lose else: print('You lose!')Sample Output:
rock, paper, scissors Game! Please enter which option you are going with: rock ('Computer picked:', 'rock') Tie Game rock, paper, scissors Game! Please enter which option you are going with: rock ('Computer picked:', 'scissors') You win
Saturday, April 7, 2012
Python: How to insert characters to the string at the end
Reference: http://stackoverflow.com/q/10059554/1276534
Credits: user1319219, Akavall, Mark Byers
Just in case you wondering what are the ways to insert character to the string at the front or back (beginning or end) of a string. Here are the ways to do it. Notice, string is immutable object, so you can not insert anymore characters into it. All we are doing is create a new string, and assign it to the variable. (That's why I did not use the same name, because I want to emphasize they are NOT the same string). But you can just as well use text = (any method)
Credits: user1319219, Akavall, Mark Byers
Just in case you wondering what are the ways to insert character to the string at the front or back (beginning or end) of a string. Here are the ways to do it. Notice, string is immutable object, so you can not insert anymore characters into it. All we are doing is create a new string, and assign it to the variable. (That's why I did not use the same name, because I want to emphasize they are NOT the same string). But you can just as well use text = (any method)
# Create a new string that have 1 x in the beginning, and 2 x at the end # So I want the string to look like this 'xHello Pythonxx' text = 'Hello Python' # Method 1, string concatenation new_text = 'x' + text + 'xx' # Method 2, create a new string with math operators i = 1 j = 2 new_text = ('x'*i) + text + ('x'*j) # Method 3, use the string's join() method # You are actually joining the 3 part into a originally empty string new_text = ''.join(('x', text, 'xx'))And in case you don't trust me and want to see the output:
xHello Pythonxx xHello Pythonxx xHello Pythonxx
Python: How to find the integer and float in a string
Reference: http://stackoverflow.com/a/10059001/1276534
Credits: MellowFellow, hexparrot
If you have a string from somewhere, say a textbook, or an input. The string contain the data information you need. How do you extract the string? There are quite a few way to do it, range from hard code accessing it from index, to finding the E=, to using regular expression. In this example we will go over the middle of the range one. Because as beginner, it might not be the best idea to introduce regular expression yet. Plus this is the version I understand, maybe the regular expression version will be provided soon. (If you can't wait, you can check out from the reference link from above, there is the regular expression solution as well.)
Credits: MellowFellow, hexparrot
If you have a string from somewhere, say a textbook, or an input. The string contain the data information you need. How do you extract the string? There are quite a few way to do it, range from hard code accessing it from index, to finding the E=, to using regular expression. In this example we will go over the middle of the range one. Because as beginner, it might not be the best idea to introduce regular expression yet. Plus this is the version I understand, maybe the regular expression version will be provided soon. (If you can't wait, you can check out from the reference link from above, there is the regular expression solution as well.)
# Assume you get this string from either a file or input from user text_string = 'The voltage is E=500V and the current is I=6.5A' # The starting index of this locating the voltage pattern voltage_begin = text_string.find('E=') # The ending index, note we are ending at 'V' voltage_end = text_string.find('V', voltage_begin) voltage_string = text_string[voltage_begin:voltage_end] print(voltage_string, 'is at index:', voltage_begin, voltage_end) #Since we know about the first to index is not useful to us voltage_string = text_string[voltage_begin+2:voltage_end] # So since now we have the exact string we want, we can cast it to an integer voltage_as_int = int(voltage_string) print(voltage_as_int)
Labels:
float,
Python,
stackoverflow goodies,
string,
Tutorial
Python: How to search a list of class element by their data (self.name) variable
Reference: http://stackoverflow.com/q/10052322/1276534
Credits: nitin, kindall
Imagine you have a class of Food, and you want to create a list that store your class of Food. How do you search your class element within your list? Take a look at the code.
Credits: nitin, kindall
Imagine you have a class of Food, and you want to create a list that store your class of Food. How do you search your class element within your list? Take a look at the code.
class Food: def __init__(self, name): self.name = name chicken = Food('Egg') cow = Food('Beef') potato = Food('French Fries') # And then you create the list of food? myFoodList = [chicken, cow, potato]The way you want to implement your class so you can search through them by their name variable is done via dictionary. (This is one way to do it, set also might work depend on your class's implementation and required functionality).
class Food: lookup = {} def __init__(self, name): self.name = name Food.lookup[name] = self chicken = Food('Egg') cow = Food('Beef') potato = Food('French Fries') # Example of a lookup from the dictionary # If your name is in your class Food's lookup dictionary if 'Egg' in Food.lookup: # Do something that you want print (Food.lookup['Egg'].name)
Friday, April 6, 2012
Python: How to remove, or pop an element randomly from a list
Reference: http://stackoverflow.com/q/10048069/1276534
Credit: Henrik, F.J, Óscar López, Niklas B.
Assuming you want to randomly remove an element from your list, you can use the random module, to generate a random number, between the valid index in your list. Take a look at the code below.
(Method 1) Let's studying this code from the inner to the outer layer.
Credit: Henrik, F.J, Óscar López, Niklas B.
Assuming you want to randomly remove an element from your list, you can use the random module, to generate a random number, between the valid index in your list. Take a look at the code below.
# Import the random module import random # This is your list with some number # But you can of course have anything you in your list myList = [1, 3, 5, 7, 9, 11] # Method #1 myList.pop(random.randrange(len(myList))) # Method #2 (This one will change your list) random.shuffle(myList) # While my list is not empty while myList: myList.pop
(Method 1) Let's studying this code from the inner to the outer layer.
- We get the length of the list, with my len(myList)
- Then we call randrange(), what randrange does it return a number that is pick randomly between the range we provide it. But except we do not actually create this list. Like range() would.
- Then finally we pop it from our list.
Subscribe to:
Posts (Atom)